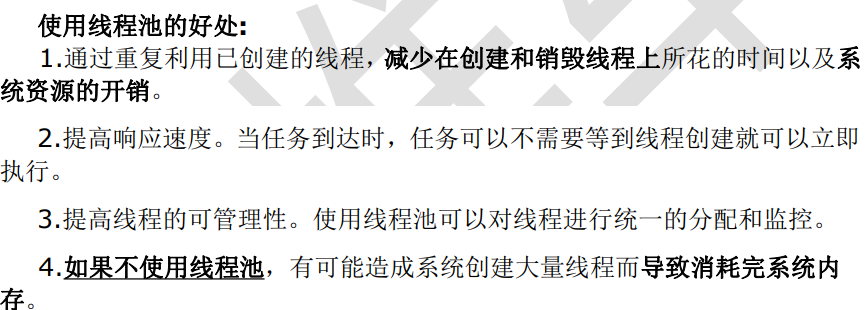
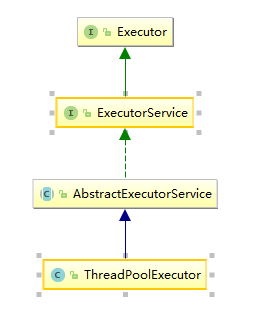
public interface Executor {
/**
* Executes the given command at some time in the future. The command
* may execute in a new thread, in a pooled thread, or in the calling
* thread, at the discretion of the {@code Executor} implementation.
*
* @param command the runnable task
* @throws RejectedExecutionException if this task cannot be
* accepted for execution
* @throws NullPointerException if command is null
*/
void execute(Runnable command);
}
就是执行任务
Executors
- java.util.concurrent.Executors#newFixedThreadPool(int)
public static ExecutorService newFixedThreadPool(int nThreads) {
return new ThreadPoolExecutor(nThreads, nThreads,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
}
返回固定数量的线程池
- java.util.concurrent.Executors#newCachedThreadPool()
public static ExecutorService newCachedThreadPool() {
return new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<Runnable>());
}
返回一个根据实际情况调整线程个数的线程池,不限制最大最大线程数量。每一个空闲线程会在60s后自动回收
- java.util.concurrent.Executors#newSingleThreadExecutor()
public static ExecutorService newSingleThreadExecutor() {
return new FinalizableDelegatedExecutorService
(new ThreadPoolExecutor(1, 1,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>()));
}
创建一个线程的线程池
- java.util.concurrent.Executors#newScheduledThreadPool(int)
public static ScheduledExecutorService newScheduledThreadPool(int corePoolSize) {
return new ScheduledThreadPoolExecutor(corePoolSize);
}
用来调度即将执行任务的线程池
我们发现都调用了 ThreadPoolExecutor
如何理解corePoolSize(核心线程数量)和maximumPoolSize(非核心线程数量)?
比如一家餐厅平时有5个厨师做菜 (corePoolSize=5)
但逢年过节来吃饭的人特别多,于是又招了3个厨师,考虑到员工成本问题,又不会招太多(maximumPoolSize=8)
当忙的几天过去了,又把新招的3个辞退掉,又恢复到corePoolSize
怎么理解阻塞队列?
分为有界队列和无界队列
1)使用有界队列时,有新任务要执行,若线程池线程数小于corePoolSize,则优先创建线程,若大于corePoolSize,把任务加入队列,
若队列已满,则在总线程数小于maximumPoolSize的情况下,创建新的线程,若线程数大于maximumPoolSize,则执行拒绝策略。
2)使用无界队列时,如LinkedBlockingQueue,任务不断入队列,直到系统资源耗尽
什么是拒绝策略?
java.util.concurrent.ThreadPoolExecutor.CallerRunsPolicy
运行当前被丢弃的任务
java.util.concurrent.ThreadPoolExecutor.AbortPolicy
直接抛出异常
java.util.concurrent.ThreadPoolExecutor.DiscardPolicy
丢弃无法处理的任务,不给予任何处理
java.util.concurrent.ThreadPoolExecutor.DiscardOldestPolicy
丢弃最老的一个请求
也可以自定义拒绝策略
除了可以使用Executors提供的线程池,也可以使用自定义线程池
说点什么
1 评论 在 "线程池"
[…] 线程池 […]