创建一个Spring Boot多模块项目
我还是用的java 8, 那用的spring boot版本也不要太高,不然会抱各种稀奇古怪版本的问题
这里spring boot我用的 2.0.0.RELEASE
创建一个新的工程,我们看到一些生成的文件:
.iml 文件、.idea目录,项目配置文件
.gitignore 文件是一个文本文件,它告诉Git 要忽略项目中的哪些文件或文件夹。 本地 .gitignore 文件通常被放置在项目的根目录中。 你还可以创建一个全局 .gitignore 文件,该文件中的所有条目都会在你所有的Git 仓库中被忽略
.mvn 用于 Maven 构建、编译、打包和部署
mvnw和mvnw.cmd(全名是maven wrapper)(属于maven技术的): 是一个常用的Maven构建工具(Maven包装器),. 它的作用类似于Gradle包装器. 它可以负责给这个特定的项目自动安装指定版本的Maven,而其他项目不受影响(其他项目采用预先设置的mavan版本)。
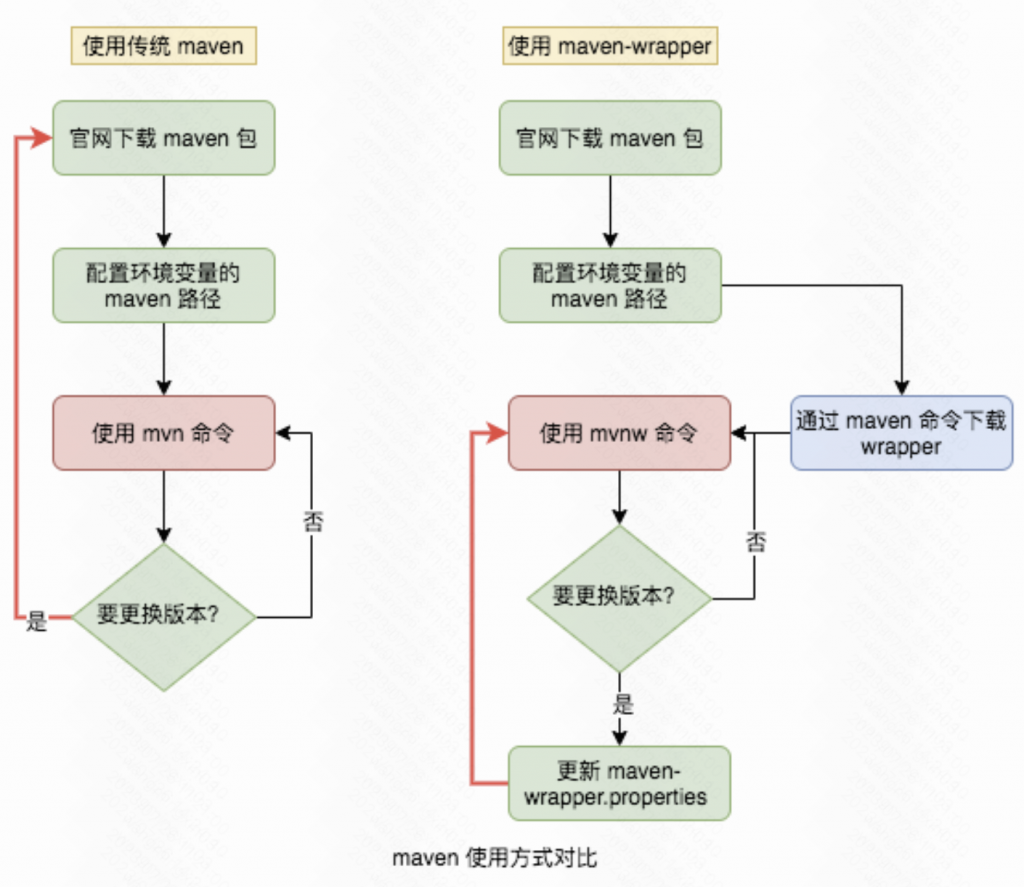
maven-wrapper 解决了应用依赖的 Maven 版本手动切换的问题,达成自动更新的目标
再创建几个子模块
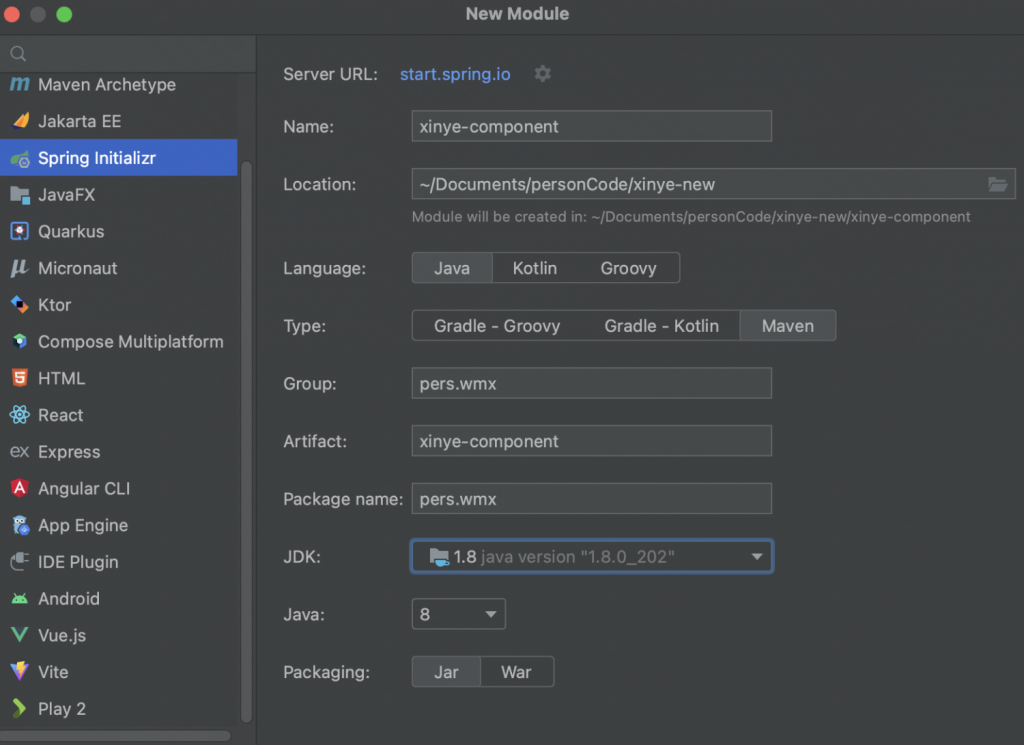
创建xinye-api, 放controller代码
xinye-component, 放业务逻辑代码
xinye-runner,定时任务、kafka消费者、rpc实现
xinye-sdk,对外暴露的rpc
我们看下父pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>pers.wmx</groupId>
<artifactId>xinye-new</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>pom</packaging>
<modules>
<module>xinye-api</module>
<module>xinye-component</module>
<module>xinye-runner</module>
<module>xinye-sdk</module>
</modules>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>pers.wmx</groupId>
<artifactId>xinye-api</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>pers.wmx</groupId>
<artifactId>xinye-component</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>pers.wmx</groupId>
<artifactId>xinye-runner</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>pers.wmx</groupId>
<artifactId>xinye-sdk</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
子模块 xinye-api pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>pers.wmx</groupId>
<artifactId>xinye-new</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>xinye-api</artifactId>
<dependencies>
<dependency>
<groupId>pers.wmx</groupId>
<artifactId>xinye-component</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
</project>
子模块 xinye-component pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>pers.wmx</groupId>
<artifactId>xinye-new</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>xinye-component</artifactId>
<packaging>jar</packaging>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<skip>true</skip>
</configuration>
</plugin>
</plugins>
</build>
</project>
子模块xinye-runner pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>pers.wmx</groupId>
<artifactId>xinye-new</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>xinye-runner</artifactId>
<dependencies>
<dependency>
<groupId>pers.wmx</groupId>
<artifactId>xinye-component</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
</project>
子模块xinye-sdk pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>pers.wmx</groupId>
<artifactId>xinye-new</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>xinye-sdk</artifactId>
<packaging>jar</packaging>
<dependencies>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<skip>true</skip>
</configuration>
</plugin>
</plugins>
</build>
</project>
工程结构:
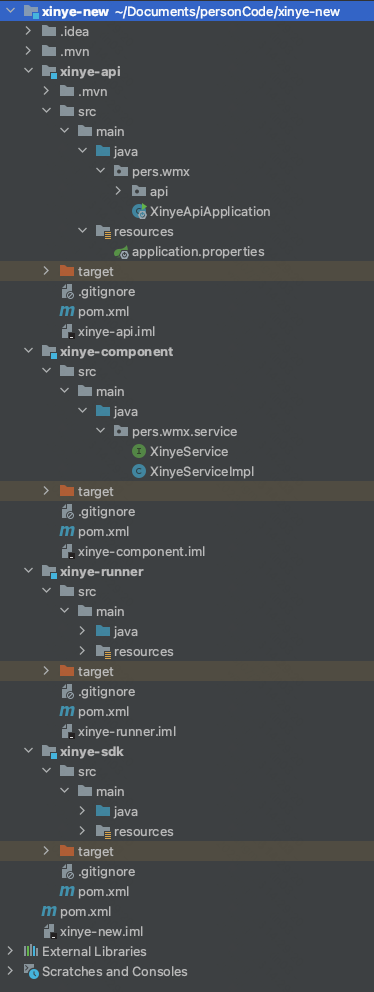
模块依赖:
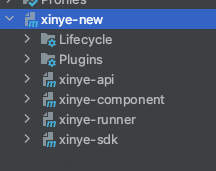
mvn clean package
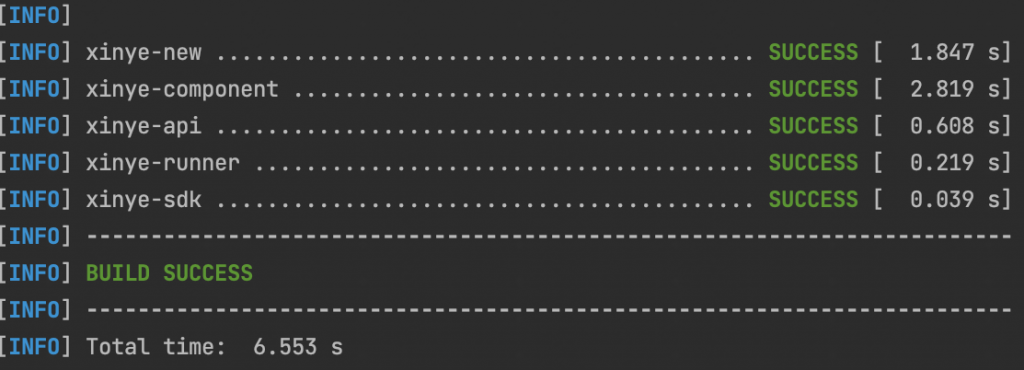
写个小demo 工程跑起来
@RestController
@RequestMapping("/test")
public class XinyeController {
@Autowired
private XinyeService xinyeService;
@RequestMapping("/test")
public Map<String, Object> test() {
Map<String, Object> result = xinyeService.get();
return result;
}
}

中间出过几次问题,Java默认选择了17,或者Spring Boot选择了高版本,都可能编译不过,或者跑不起来
遇到过以下报错:
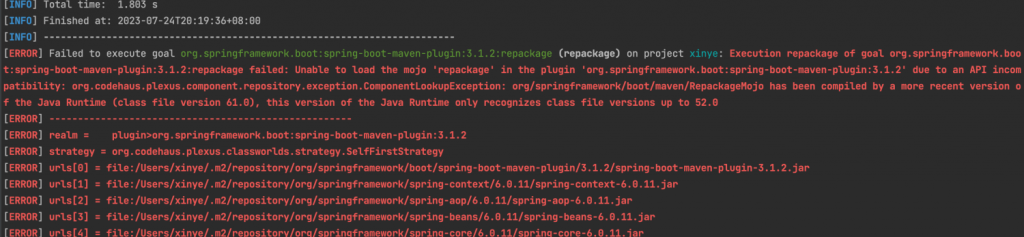

还有一些稀奇古怪的报错,都是因为上述说的版本问题
然后工程中的一些java版本都统一下
settings
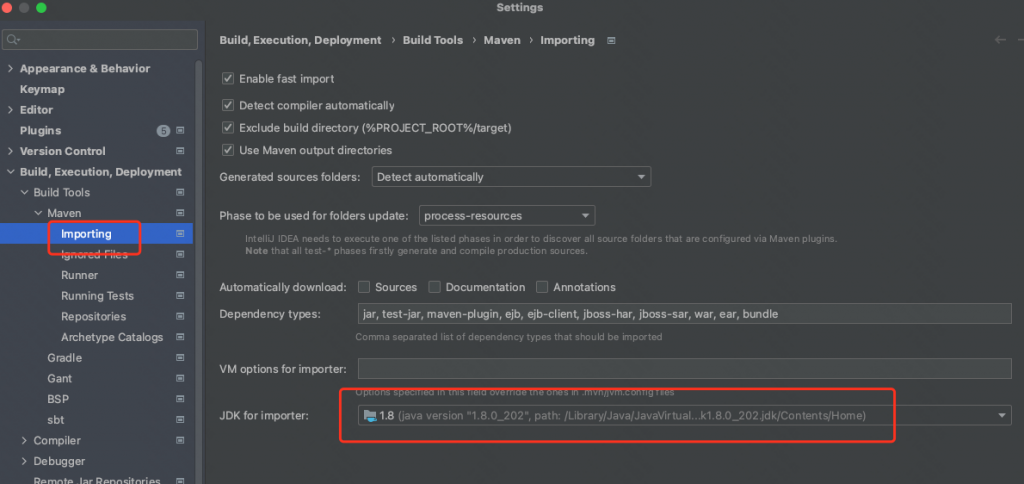
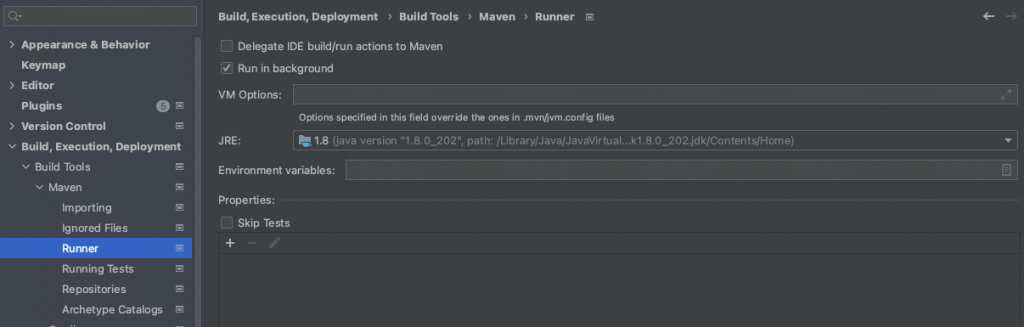
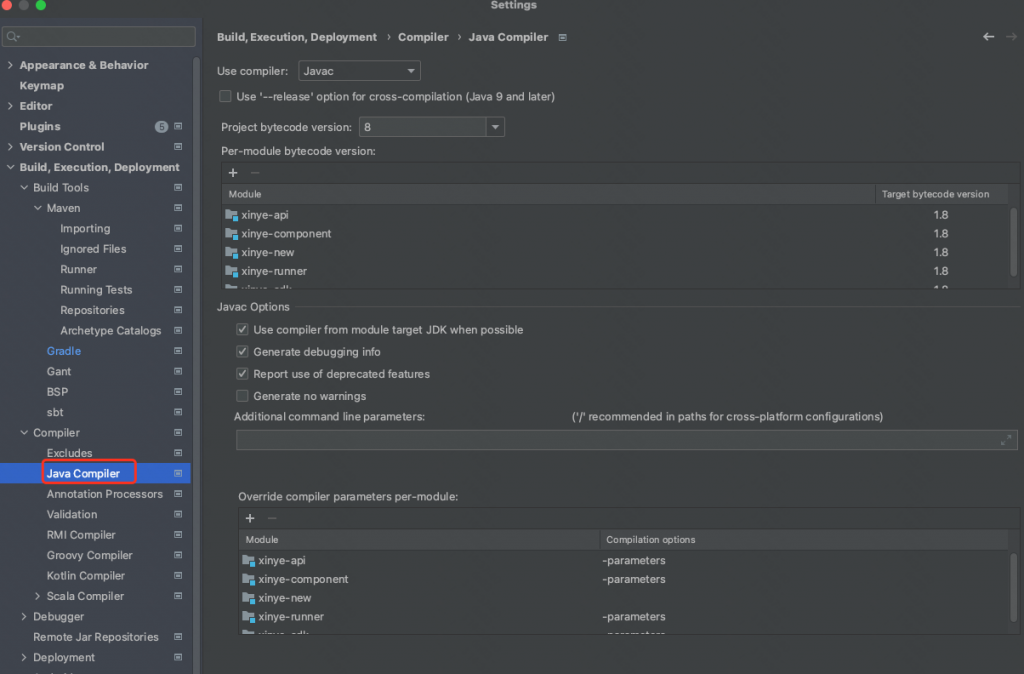
project-structure
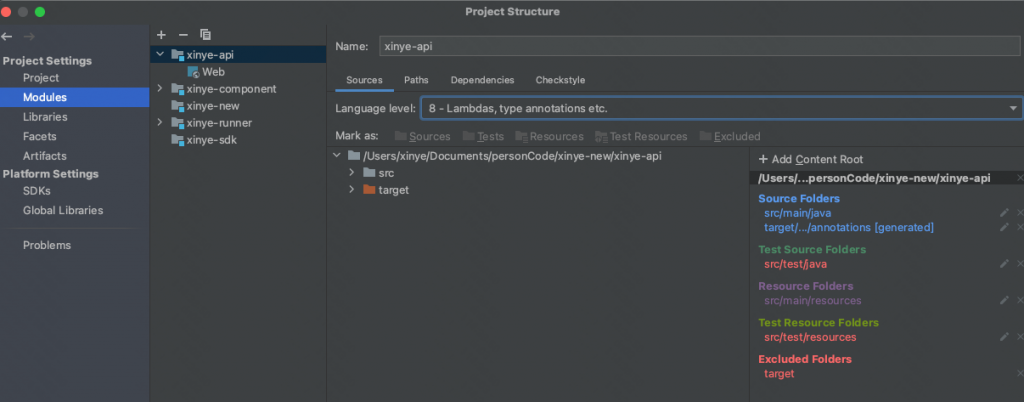
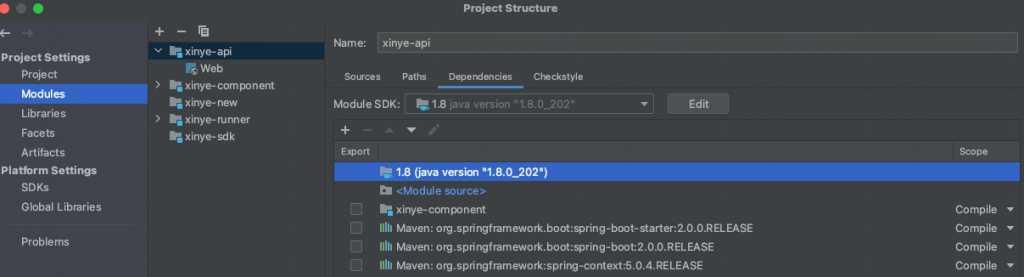
最后把本地新建的工程推到远端吧
创建一个新的仓库
然后本地依次执行
git init
git add README.md
git commit -m "first commit"
git branch -M main
git remote add origin git@github.com:xinyeshuaiqi/xinye-new.git
git push -u origin main
转载请注明:汪明鑫的个人博客 » 创建Spring Boot maven多模块项目
说点什么
您将是第一位评论人!